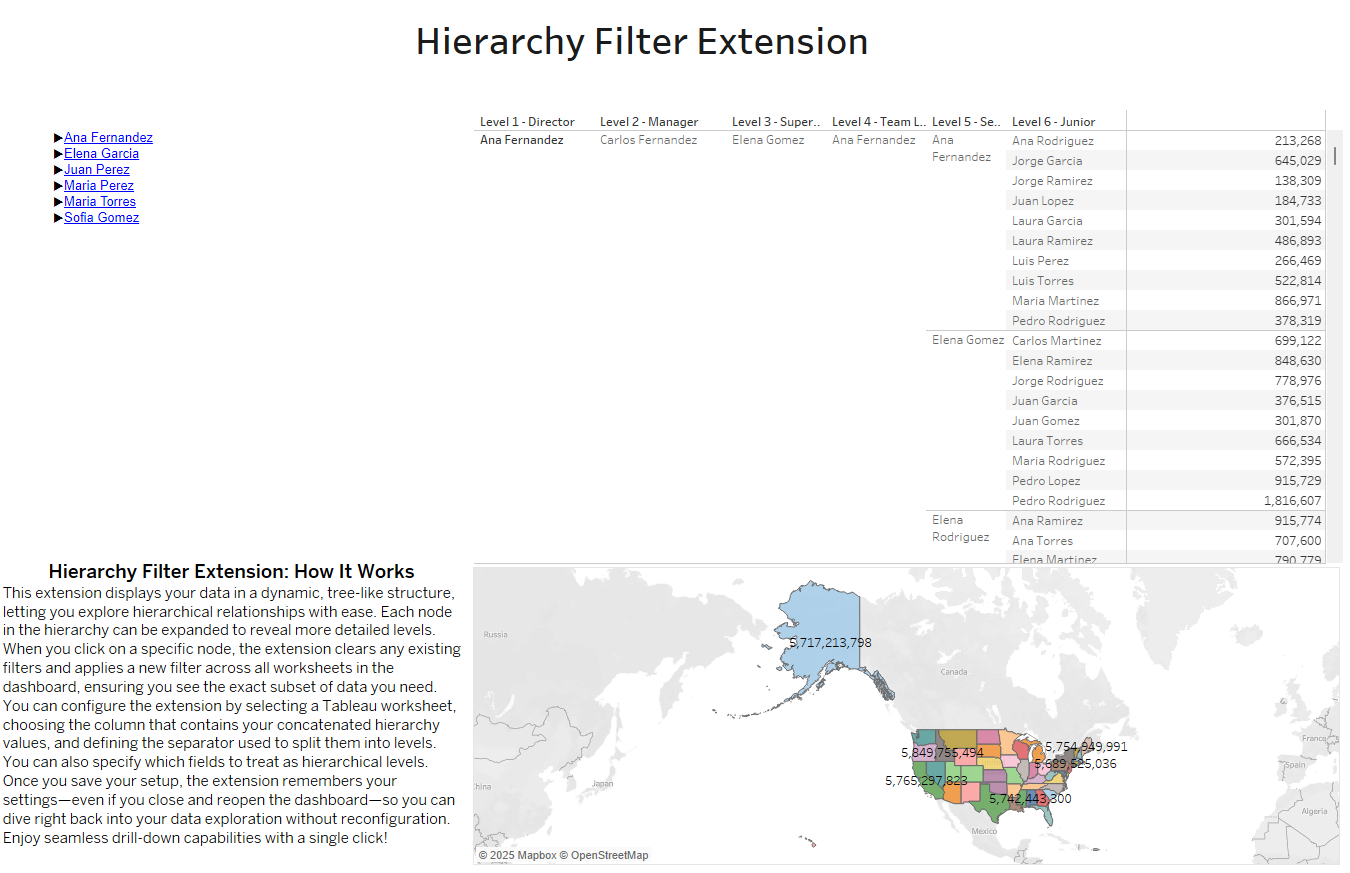
Supercharge Your Tableau Dashboards: Build a Powerful Hierarchical Filter Plugin with Vue.js
Supercharge Your Tableau Dashboards: Build a Powerful Hierarchical Filter Plugin with Vue.js
Introduction
Are you struggling with Tableau’s default filtering options when dealing with complex, multi-level data? A Hierarchical Filter Plugin can completely transform how you navigate and analyze structured data in your dashboards.
In this guide, I’ll walk you through the development of a custom Tableau extension using Vue.js and the Tableau Extensions API. This plugin enables dynamic filtering through an interactive tree structure, making it perfect for analyzing organizational structures, product hierarchies, geographical data, and more.
Why You Need a Hierarchical Filter in Tableau
Tableau’s native filters are powerful, but they can be limiting when dealing with data that has multiple levels of hierarchy. Instead of manually selecting individual filters across different levels, wouldn’t it be great to click through an intuitive tree structure to filter your data dynamically?
This plugin provides exactly that. With it, you can: ✅ Visualize hierarchical data in an interactive, expandable tree.
✅ Apply filters dynamically based on your selections.
✅ Enhance dashboard usability, making data exploration easier and faster.
✅ Save and persist settings, so you don’t have to reconfigure filters every time you open Tableau.
Tech Stack: What Powers This Plugin?
This Tableau extension is built using: ✅ Vue.js – For a smooth, interactive UI.
✅ Tableau Extensions API – To seamlessly integrate with Tableau’s filtering system.
✅ JavaScript & HTML – For structure and logic.
How the Hierarchical Filter Works
The plugin loads data from a selected worksheet in Tableau and constructs an interactive tree structure. Users can then click on any node in the hierarchy to apply filters dynamically.
Below is the core structure of index.html
, which sets up the configuration panel, the hierarchical tree, and the filtering logic.
index.html
<button
v-if="showConfigButton"
@click="openConfiguration"
class="config-button"
>
Configure Hierarchical Filter
</button>
<div v-if="treeData && Object.keys(treeData).length" class="hierarchy-tree">
<ul>
<tree-node
v-for="(children, node) in treeData"
:key="node"
:node="node"
:children="children"
:path="[]"
:levels="selectedHierarchyFields"
@filter="applyHierarchyFilter"
></tree-node>
</ul>
</div>
What This Code Does:
- Displays a configuration button only when the plugin hasn’t been set up.
- Generates a tree view from Tableau’s worksheet data.
- Each node triggers a filter event dynamically when selected.
Saving and Persisting User Settings
A key challenge in developing this plugin was ensuring that users don’t have to set it up every time they open Tableau. To solve this, we use Tableau Extensions API to store and retrieve user settings.
Saving Configuration Settings (script.js)
saveConfiguration() {
console.log("Configuration Saved =>", {
worksheet: this.selectedWorksheet,
idColumn: this.selectedIDColumn,
separator: this.separator,
hierarchyFields: this.selectedHierarchyFields
});
this.showConfig = false;
// Store configuration data
const extensionData = {
configComplete: true,
worksheet: this.selectedWorksheet,
idColumn: this.selectedIDColumn,
separator: this.separator,
hierarchyFields: this.selectedHierarchyFields
};
tableau.extensions.settings.set("data", JSON.stringify(extensionData));
// Save settings asynchronously
tableau.extensions.settings.saveAsync().then(() => {
console.log("✅ Settings saved successfully!");
this.showConfigButton = false;
this.loadHierarchy();
}).catch(error => console.error("❌ Error saving settings:", error));
}
This function ensures that:
- User preferences persist across sessions.
- Filters are applied instantly after saving settings.
- The configuration button disappears once the setup is complete.
The Impact: A More Interactive & Efficient Tableau Dashboard
By integrating a Hierarchical Filter Plugin, your Tableau dashboards become more intuitive, dynamic, and user-friendly. No more navigating through long dropdown lists or manually setting filters – with just a few clicks, you can drill down into your data effortlessly.
Want to Implement This in Your Dashboard?
If you’re interested in using this plugin or customizing it for your needs, feel free to contact me at:
📩 contact@albertcardenas.com
I’d love to hear how you’re using Tableau and help optimize your dashboards with custom solutions! 🚀